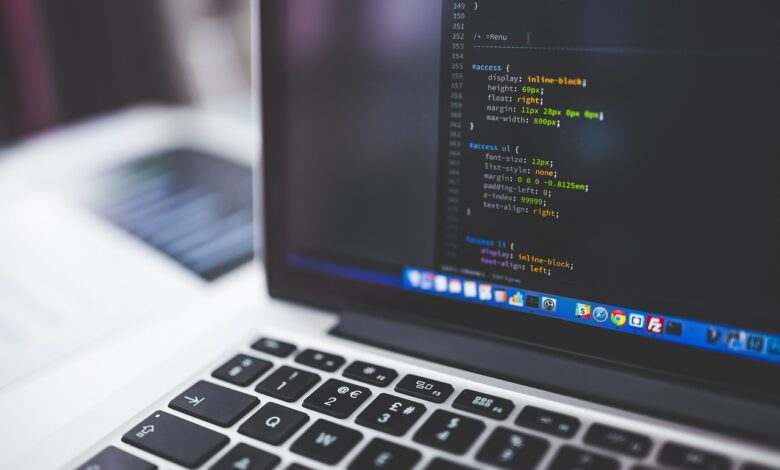
Learning how to code has become an essential skill in today’s tech-driven world. Whether you’re looking to pursue a career in software development or simply interested in automating tasks, building websites, or analyzing data, understanding the basics of coding is an excellent starting point. This tutorial is designed for beginners who have no prior experience with programming and want to learn the fundamentals. By the end of this guide, you’ll have a solid foundation to start coding on your own.
Why Learn Coding?
Before diving into the technical aspects, it’s essential to understand why learning to code is valuable. Coding opens the door to endless possibilities, from creating apps and websites to developing games, automating daily tasks, or even analyzing large sets of data. Learning to code enhances problem-solving skills, encourages logical thinking, and provides a gateway to understanding how modern technologies work. Additionally, coding offers excellent career opportunities in various fields, including technology, finance, healthcare, education, and more.
Choosing the Right Programming Language
One of the most common questions beginners ask is, “Which programming language should I start with?” The answer depends on your goals and interests. Here’s a brief overview of some of the most beginner-friendly programming languages:
- Python: Known for its simplicity and readability, Python is a great starting language. It’s widely used in web development, data science, artificial intelligence, and automation.
- JavaScript: If you’re interested in web development, JavaScript is a must. It’s the backbone of most modern websites and enables you to create dynamic content and interactive user experiences.
- HTML/CSS: Though technically not programming languages, HTML and CSS are essential for front-end web development. HTML structures the content of web pages, while CSS styles it.
- Scratch: Designed for younger learners or complete beginners, Scratch uses a visual interface with drag-and-drop blocks to help users understand coding logic without the complexities of syntax.
Setting Up Your Development Environment
Before you can start coding, you need to set up your development environment. Here are the basic tools you’ll need:
- A Code Editor: A code editor is where you’ll write your code. Some popular choices include:
- Visual Studio Code: A powerful, free editor with various extensions and support for multiple programming languages.
- Sublime Text: Lightweight and user-friendly, Sublime Text is great for beginners.
- Atom: Another free editor that is highly customizable and beginner-friendly.
- Compilers/Interpreters: Depending on the language you choose, you may need a compiler or interpreter to run your code. For Python, for example, you can download the interpreter from Python’s official website.
- A Web Browser: If you’re working with HTML, CSS, or JavaScript, a browser like Chrome, Firefox, or Safari will allow you to see the results of your code.
- Version Control (Optional for Beginners): As you progress, learning version control systems like Git will be useful for managing and collaborating on projects.
Basic Concepts of Coding
1. Syntax
Syntax refers to the rules that define the structure of a programming language. Just like grammar in human languages, every programming language has its own syntax that must be followed. For example, in Python, you might write:
print("Hello, World!")
In JavaScript, the same code looks like:
console.log("Hello, World!");
Each language has unique syntax rules, but many programming concepts are universal.
2. Variables
Variables are used to store data. They can hold numbers, text, or other types of data, depending on the language. Here’s an example in Python:
x = 5
y = "Hello"
In this case, x
is an integer, and y
is a string (text).
3. Data Types
Data types represent the kind of data you’re working with. Common data types include:
- Integers: Whole numbers (e.g., 5, -3)
- Floats: Decimal numbers (e.g., 4.5, -2.3)
- Strings: Text (e.g., “Hello”, “World”)
- Booleans: True or False values (e.g., True, False)
4. Conditionals
Conditionals allow you to execute certain pieces of code based on specific conditions. Here’s a basic example in Python:
if x > 0:
print("x is positive")
else:
print("x is not positive")
In this example, the program checks whether the variable x
is greater than 0, and prints the corresponding message.
5. Loops
Loops allow you to repeat a block of code multiple times. There are two common types of loops:
- For Loop: Repeats a block of code a specific number of times.
for i in range(5):
print(i)
- While Loop: Continues to execute the block of code as long as a condition is true.
while x > 0:
print(x)
x -= 1
6. Functions
Functions allow you to reuse blocks of code. You define a function once, and then you can call it whenever you need it. Here’s a simple example in Python:
def greet():
print("Hello, World!")
greet()
In this example, the function greet()
is defined once and then called to print the message “Hello, World!”
Writing Your First Program
Let’s walk through writing a simple program in Python. This program will ask for the user’s name and greet them.
# Asking for user input
name = input("What is your name? ")
# Printing a greeting message
print(f"Hello, {name}! Welcome to coding.")
In this code, the input()
function takes input from the user, and the print()
function outputs a greeting message that includes the user’s name.
Debugging and Testing Your Code
As a beginner, you will undoubtedly run into errors when coding. Debugging is the process of finding and fixing these errors. Here are some common types of errors:
- Syntax Errors: These occur when you violate the syntax rules of the programming language. For example, forgetting to close parentheses or missing a colon in Python.
- Logic Errors: The program runs, but it doesn’t produce the expected result due to a mistake in the logic. For example, using
>
when you meant to use<
. - Runtime Errors: These errors occur while the program is running. They might be caused by dividing by zero or trying to access an element in a list that doesn’t exist.
Coding Best Practices for Beginners
As you progress, it’s essential to follow good coding practices to make your code more readable and maintainable. Here are a few tips:
- Comment Your Code: Use comments to explain what your code does. This is helpful for both you and others who may read your code.
- Keep Your Code Clean and Organized: Write code in small, manageable blocks (e.g., functions) instead of one large block of code.
- Use Meaningful Variable Names: Instead of naming variables
x
andy
, use descriptive names likeage
oruserName
to make your code easier to understand. - Test Your Code Regularly: Don’t wait until you finish your entire program to test it. Test small parts of your code as you write them.
Next Steps: Expanding Your Coding Skills
Once you’ve mastered the basics, you can start expanding your skills. Here are a few ways to grow:
- Build Small Projects: Start by building simple projects like a calculator, to-do list, or a personal website. These will help reinforce what you’ve learned.
- Learn About Object-Oriented Programming (OOP): OOP is a more advanced concept that involves creating objects to model real-world problems. Python, Java, and C++ are great languages for learning OOP.
- Explore Data Structures and Algorithms: As you advance, you’ll need to understand how data is stored and manipulated efficiently. Learning about arrays, linked lists, stacks, queues, and algorithms will take your coding skills to the next level.
- Join Coding Communities: Being part of a community can help you learn faster and stay motivated. Platforms like GitHub, Stack Overflow, and Reddit have active coding communities where you can ask questions and collaborate on projects.